Connectivity Manager for Unity Android
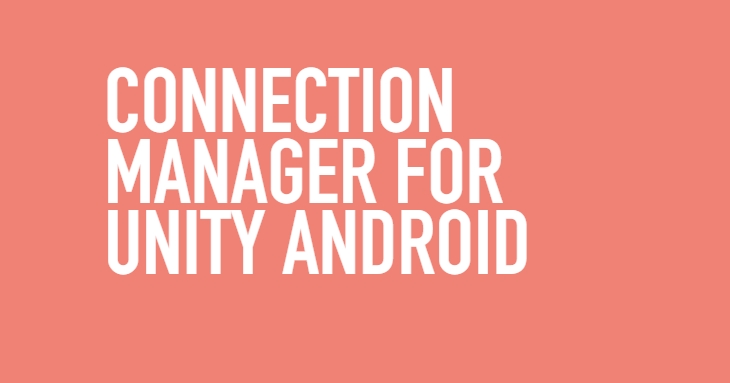
This package let’s you listen for connectivity changes on your Android device.
Basic functionallity:
- Detect when the device is connected to a network
- Detect when the network connection is lost.
- Receive updates when the network’s capabilities are updated ( wifi, cellular, ethernet, signal strength and etc )
- Download the package from Package Manager
- Import it in your project
In order to start listening for connectivity changes you should first register a listener using:
// Let's say in your script's onStart()
ConnectionManager.RegisterListener();
Subscribe for the events depending on what you need:
private void OnEnable()
{
// When the device has actual internet connection.
ConnectionManager.OnConnectedToInternet += OnConnectedToInternet;
// When the device is connected to a network. That doesn't mean that
// the device has internet connection.
ConnectionManager.OnNetworkAvailable += OnNetworkAvailable;
// When the device is no longer connected to a network.
ConnectionManager.OnNetworkLost += OnNetworkLost;
// When network's capabilities are updated.
ConnectionManager.OnCapabilitiesChanged += OnCapabilitiesChanged;
}
private void OnDisable()
{
// Don't forget to unsubscribe from the events.
ConnectionManager.OnConnectedToInternet -= OnConnectedToInternet;
ConnectionManager.OnNetworkAvailable += OnNetworkAvailable;
ConnectionManager.OnNetworkLost -= OnNetworkLost;
ConnectionManager.OnCapabilitiesChanged -= OnCapabilitiesChanged;
}
private void Start()
{
// Register connection listener
ConnectionManager.RegisterListener();
}
private void OnDestroy()
{
// Remove the listener
ConnectionManager.UnregisterListener();
}
private void OnNetworkAvailable()
{
ConnectionManager.CheckInternetConnection();
}
private void OnNetworkLost()
{
// The device is no longer connected to a network
}
private void OnConnectedToInternet(bool isConnected)
{
// If isConnected == true , the device has an access to internet,
// otherwise not.
}
private void OnCapabilitiesChanged(ConnectionInfo info)
{
// Network's capabilities are updated / changed.
}
OnNetworkAvailable is fired when the device is connected to a network, but this doesn’t mean that it’s actually connected to an internet. Easy way to simulate this is let’s say connect to a Wifi Hotspot created from another Android device which is not connected to a cellular network for example. Your device will be connected to a network without active internet connection. In order to check if there is an active connection to the internet you can call:
ConnectionManager.CheckInternetConnection();
Which pings Google’s servers ( because if www.google.com is not reachable that means the end of worlds is near :D ) to determine if the device is connected to internet. And the result of that call is returned using:
ConnectionManager.OnConnectedToInternet // event
You can check the isConnected variable to determine the state of the connection.
And last but not least VERY IMPORTANT - DO NOT FORGET to update your AndroidManifest.xml file and include those two permissions:
<uses-permission android:name="android.permission.CHANGE_NETWORK_STATE" />
<uses-permission android:name="android.permission.INTERNET" />
And you are all good to go!
If you have any questions please do not hesitate to contact me using support@hardartcore.com